10 JavaScript Tricks Every Developer Should Know
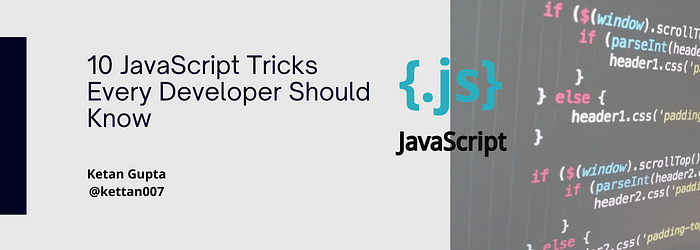
JavaScript is a versatile and dynamic programming language that powers much of the web. Whether you’re building a small web application or a complex single-page app, you need to know the best tricks that the language has to offer. In this article, we’ll cover 10 of the most important JavaScript tricks that every developer should know.
1. Ternary Operator:
The ternary operator allows you to write an if-else statement in a single line, making your code more concise and readable. Instead of writing a traditional if-else statement, you can use the ternary operator to write the same logic in a more concise way.
let x = 10;
let result = x > 0 ? 'positive' : 'negative';
console.log(result); // 'positive'
2. Destructuring:
Destructuring is a feature in JavaScript that allows you to extract values from objects and arrays and assign them to variables with a concise syntax. This makes it easy to work with complex data structures and can greatly improve the readability of your code.
let user = { name: 'John', age: 30 };
let { name, age } = user;
console.log(name); // 'John'
console.log(age); // 30
3. Arrow Functions:
Arrow functions are a shorthand for writing anonymous functions, making your code more concise and readable. Arrow functions also have a more concise syntax and have different behavior for the this
keyword, making them ideal for use in callbacks and event handlers.
let numbers = [1, 2, 3, 4, 5];
let square = numbers.map(x => x * x);
console.log(square); // [1, 4, 9, 16, 25]
4. Spread Operator
The spread operator allows you to spread elements of an array into a new array, a new object, or even pass elements as separate arguments to a function. This makes it easy to work with arrays and objects, and can greatly improve the readability of your code.
let numbers = [1, 2, 3];
let moreNumbers = [4, 5, 6];
let allNumbers = [...numbers, ...moreNumbers];
console.log(allNumbers); // [1, 2, 3, 4, 5, 6]
5. Array.map()
The map() method is a powerful array function that allows you to transform each element in an array and return a new array. This makes it easy to work with arrays and can greatly improve the readability of your code.
let numbers = [1, 2, 3, 4, 5];
let square = numbers.map(x => x * x);
console.log(square); // [1, 4, 9, 16, 25]
6. Array.filter()
The filter() method is a powerful array function that allows you to select elements from an array based on a condition and return a new array. This makes it easy to work with arrays and can greatly improve the readability of your code.
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(x => x % 2 === 0);
console.log(evenNumbers); // [2, 4]
7. Array.reduce()
The reduce() method is a powerful array function that allows you to reduce an array to a single value by iterating over each element and accumulating a result. This makes it easy to perform operations like summing, averaging, or concatenating arrays and can greatly improve the readability of your code.
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((acc, x) => acc + x, 0);
console.log(sum); // 15
8. Object.keys() and Object.values()
The keys() and values() methods allow you to extract the keys and values of an object, respectively and return them as arrays. This makes it easy to work with objects and can greatly improve the readability of your code.
let user = { name: 'John', age: 30 };
let keys = Object.keys(user);
let values = Object.values(user);
console.log(keys); // ['name', 'age']
console.log(values); // ['John', 30]
9. Template Literals
Template literals are a new way of writing string literals in JavaScript, allowing you to embed expressions and variables directly into the string. This makes it easy to work with strings and can greatly improve the readability of your code.
let name = 'John';
let message = `Hello, ${name}!`;
console.log(message); // 'Hello, John!'10. Higher-Order Functions
Also Read: JSON Web Token (JWT) in node.js (Implementing using Refresh token)
10. Higher-order functions are functions that take other functions as arguments or return functions as values. This makes it easy to write reusable and composable code, and can greatly improve the readability of your code.
let numbers = [1, 2, 3, 4, 5];
let double = numbers.map(x => x * 2);
let sum = double.reduce((acc, x) => acc + x, 0);
console.log(sum); // 30
In conclusion, these are just some of the many JavaScript tricks that every developer should know. By mastering these tricks, you can write cleaner, more readable, and more efficient code, and become a more effective and productive JavaScript developer.